Basic routing & views
Basic routing
- The file routes/web.php contains all the routes that you want to visit (or get) through a browser
- When a user visits the home page http://vinyl_shop.test of the application (indicated by
'/'
), the Laravel 'welcome' view is shown
Route::get('/', function () {
return view('welcome');
});
1
2
3
2
3
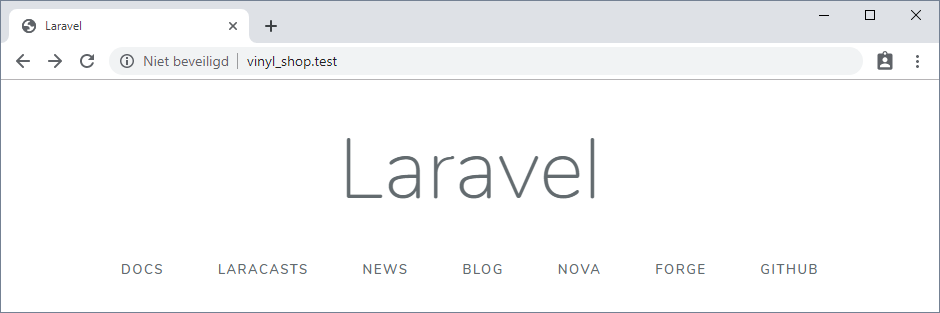
REMARK
- In this first piece of code, we already encounter facades and helper functions, two very important features of Laravel
- In the statement
Route::get(...)
, it looks like a static methodget()
of a classRoute
is called. However,Route
is not an ordinary PHP class, but a Laravel facade.
Facades are special Laravel classes that provide easy access to lots of Laravel features with static method calls. A detailed understanding of this architectural concept is beyond the scope of this course. Remember for now that a facade acts like a proxy: when the statementRoute::get(...)
is executed, an object of a specific class linked to theRoute
facade (in this case \vendor\laravel\framework\src\Illuminate\Routing\Router.php) is initiated behind the scenes, and the methodget()
is called (on this object) in a non-static way. - The
view()
function is one of the numerous Laravel helper functions. The function retrieves an instance of the view specified in the function argument.
- In the statement
- Change the code and the project home page gets adjusted
Route::get('/', function () {
//return view('welcome');
return 'The Vinyl Shop';
});
1
2
3
4
2
3
4
- Add a new route to the URL http://vinyl_shop.test/contact-us and show some contact information
Route::get('contact-us', function () {
return 'Contact info';
});
1
2
3
2
3

Basic views
- Views correspond to the HTML (styled with CSS) that gets rendered in the browser
- Views are stored in the folder resources/views
- The view 'welcome' (called in the route to the home page) corresponds to the file welcome.blade.php
- Blade is the templating engine (shipped with Laravel) that will be covered (extensively) further on in this course
NAMING CONVENTIONS
All views in Laravel must have the extension .blade.php
- Make a new view contact.blade.php in the folder resources/views with some basic HTML code
<h1>Contact info</h1>
<p>The Vinyl Shop</p>
<p><a href="mailto:info@thevinylshop.com">info@thevinylshop.com</a></p>
1
2
3
4
2
3
4
- Adjust the 'contact-us' route in the file routes/web.php
Route::get('contact-us', function () {
//return 'Contact info';
return view('contact');
});
1
2
3
4
2
3
4
REMARK
Do not include the extension .blade.php in the parameter of the view()
function
- When the URL vinyl_shop.test/contact-us is visited, the view contact.blade.php is shown
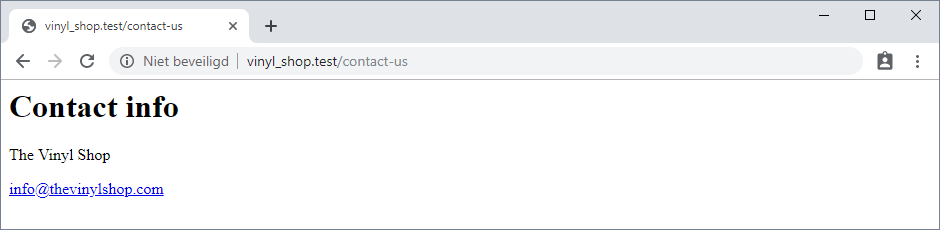
Single line notation
- The code
Route::get('contact-us', function () {
return view('contact');
});
1
2
3
2
3
can be replaced by
Route::view('contact-us', 'contact');
1
EXERCISE: Refactor home page
- Create a new home page home.blade.php (with a
h1
and ap
) - Refactor the route to it with a single line notation
Route::view('/', 'home');
Route::view('contact-us', 'contact');
1
2
2
Organizing views in subfolders
- It's best practice to group the views of a project in subfolders
- Make a subfolder admin/records in resources/views and add a new view index.blade.php in this subfolder
<h1>Records</h1>
<ul>
<li>Record 1</li>
<li>Record 2</li>
<li>Record 3</li>
</ul>
1
2
3
4
5
6
7
2
3
4
5
6
7
- Add a new route 'admin/records' (URL = http://vinyl_shop.test/admin/records) to this view in the file routes/web.php
Route::get('admin/records', function (){
return view('admin.records.index');
});
1
2
3
2
3
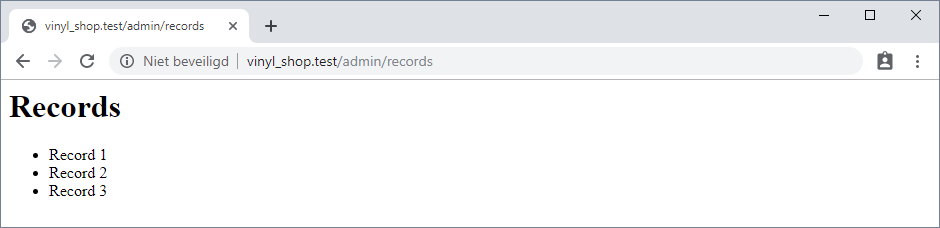
REMARKS
- We use the extended
Route::get()
notation here as we want to pass some data to this view in the next section, which is impossible with the single line notation - The view can also be returned with the statement
return view('admin/records/index')
, in which a/
is used instead of a.
to navigate through the folder structure of the views
Passing data to the views
- Create an array
$records
with some data in the 'admin/records' route - The
view()
function can receive an associative array as its second argument, through which this data can be passed to the view
Route::get('admin/records', function (){
$records = [
'Queen - Greatest Hits',
'The Rolling Stones - Sticky Fingers',
'The Beatles - Abbey Road'
];
return view('admin.records.index', [
'records' => $records
]);
});
1
2
3
4
5
6
7
8
9
10
11
2
3
4
5
6
7
8
9
10
11
- In the view index.blade.php, the key of this associative array can be used to access the data
<ul>
<?php
foreach ($records as $record){
echo "<li> $record </li>";
//echo '<li>' . $record . '</li>';
}
?>
</ul>
1
2
3
4
5
6
7
8
2
3
4
5
6
7
8
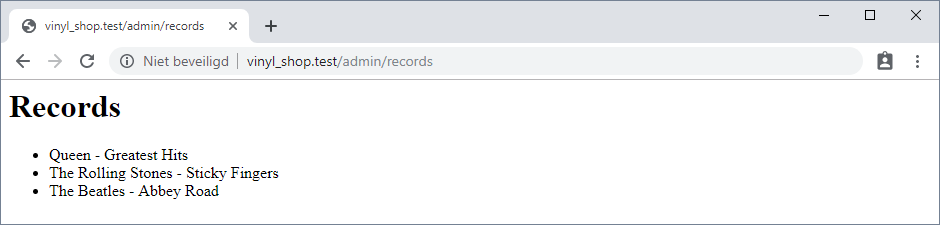
REMARK
Notice that it is the key of the array that must be used to access the data in the view. If you would write
return view('admin.records.index', [
'someSillyName' => $records
]);
1
2
3
2
3
in the route, the view must be adjusted accordingly
<ul>
<?php
foreach ($someSillyName as $record){
echo "<li> $record </li>";
//echo '<li>' . $record . '</li>';
}
?>
</ul>
1
2
3
4
5
6
7
8
2
3
4
5
6
7
8
Blade syntax: @foreach and double curly braces {{ }}
- Using Blade, the
foreach
loop in the view index.blade.php can be simplified
<ul>
@foreach ($records as $record)
<li>{{ $record }}</li>
@endforeach
</ul>
1
2
3
4
5
2
3
4
5
- Blade-specific syntax always starts with
@
- The PHP
foreach
loop is replaced by a Blade@foreach
loop- Ends with
@endforeach
/ no surrounding braces{ }
- The (body of the) loop contains HTML and PHP
- Data passed to the view can be displayed by wrapping this data within double curly braces
{{ }}
- Ends with
REMARKS
- Blade
{{ }}
statements automatically escape (any HTML in) the data to prevent cross-site scripting (XSS) attacks
$records = [
'Queen - <b>Greatest Hits</b>',
'The Rolling Stones - <em>Sticky Fingers</em>',
'The Beatles - Abbey Road'
];
1
2
3
4
5
2
3
4
5
@foreach ($records as $record)
<li>{{ $record }}</li>
@endforeach
1
2
3
2
3
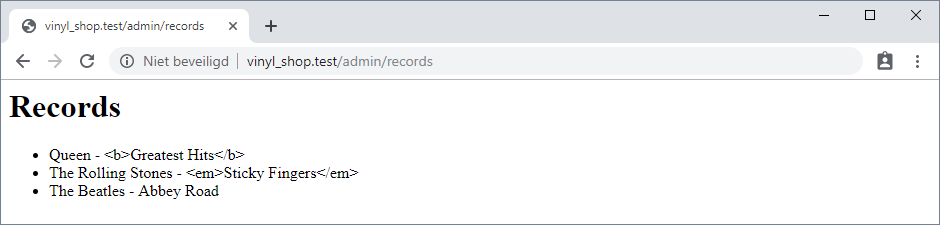
- Use
{!! !!}
if unescaped, raw output is needed
@foreach ($records as $record)
<li>{!! $record !!}}</li>
@endforeach
1
2
3
2
3
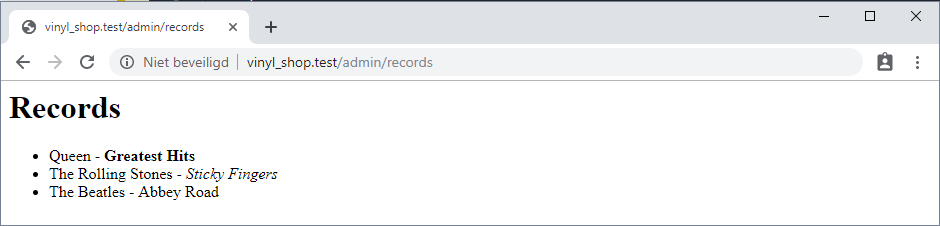
Route groups
- Route groups allow you to share route attributes, such as middleware (later in this course) across all the routes within the group
- The
prefix()
method can be used to prefix each route in the group with a given URI - Combine all routes, starting from http://vinyl_shop.test/admin/ in a group with the prefix admin
Route::prefix('admin')->group(function () {...})
// Old version
/*
Route::get('admin/records', function (){...});
*/
// New version with prefix and group
Route::prefix('admin')->group(function () {
Route::get('records', function (){
$records = [
'Queen - Greatest Hits',
'The Rolling Stones - Sticky Fingers',
'The Beatles - Abbey Road'
];
return view('admin.records.index', [
'records' => $records
]);
});
});
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
- Open http://vinyl_shop.test/admin/records in a browser
- Now open http://vinyl_shop.test/admin/ in a browser
- This results in a 404 error because the route does not exist
- You can easily solve this by redirecting the URL http://vinyl_shop.test/admin/ to an existing page like http://vinyl_shop.test/admin/records
Route::prefix('admin')->group(function () {
Route::redirect('/', 'records');
Route::get('records', function (){...});
});
1
2
3
4
2
3
4